常用汇编指令
lea 加载有效地址
lea eax, [ebx+8] 将ebx+8存储的内存地址 +8 传给eax
mov eax, [ebx+8] 将ebx+8内存地址指向的数据 传给eax
cmp
算数运算
sub 减
add 加
inc +1
dec -1
mul 乘
div 除
条件指令及跳转指令
https://blog.csdn.net/counsellor/article/details/81005101
jmp 无条件跳转 p74-76
这几个都是根据标志位跳转
jz 如果ZF标志位置位则跳转 等于
jnz 如果ZF标志位被清除则跳转 不等于
je 等于跳转
jg 有符号大于则跳转
test eax,eax 检测目标值是否为0 ,即两个操作数按位与运算,为0的话设置标志位ZF为1,否则为0(不修改使用的操作数)
cmp eax,ebx 如果两个参数相等,ZF标志位置位
一般在cmp指令后用je,test指令后用jz
函数使用的
call
leave
enter
ret
https://www.codenong.com/10483544/
全局变量与局部变量
1 2 3 4 5 6 7 8 9 10 11
| #include <stdio.h>
int i = 1;
int main() { int j =2; printf("this is:%d %d\n",i,j); return 0;
}
|

全局变量i通过内存地址引用,局部变量通过栈地址引用
算数运算
1 2 3 4 5 6 7 8 9 10 11 12 13
| #include <stdio.h>
int main() { int i = 10; int j=2; int k; i = i +2; k = i/j; printf("this is:%d %d %d\n",i,j,k); return 0;
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| .text:000000000000064A push rbp .text:000000000000064B mov rbp, rsp .text:000000000000064E sub rsp, 10h .text:0000000000000652 mov [rbp+var_C], 0Ah ; int i =10 .text:0000000000000659 mov [rbp+var_8], 2 ;int j=2; .text:0000000000000660 add [rbp+var_C], 2 ; i = i +2; .text:0000000000000664 mov eax, [rbp+var_C] ; 把i放到eax中当作被除数 .text:0000000000000667 cdq .text:0000000000000668 idiv [rbp+var_8] ;j是除数 .text:000000000000066B mov [rbp+var_4], eax ;结果放到k中 .text:000000000000066E mov ecx, [rbp+var_4] ;k .text:0000000000000671 mov edx, [rbp+var_8] ;j .text:0000000000000674 mov eax, [rbp+var_C] ;i .text:0000000000000677 mov esi, eax ;i .text:0000000000000679 lea rdi, format ; "this is:%d %d %d\n"
|
识别if语句
• if语句的结构
– if语句主要由判断语句和跳转语句构成
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| #include <stdio.h>
int main() { int i = 3;
if(i == 3) { printf(" this is 3"); }else{ printf(" this is not 3"); }
return 0;
}
|
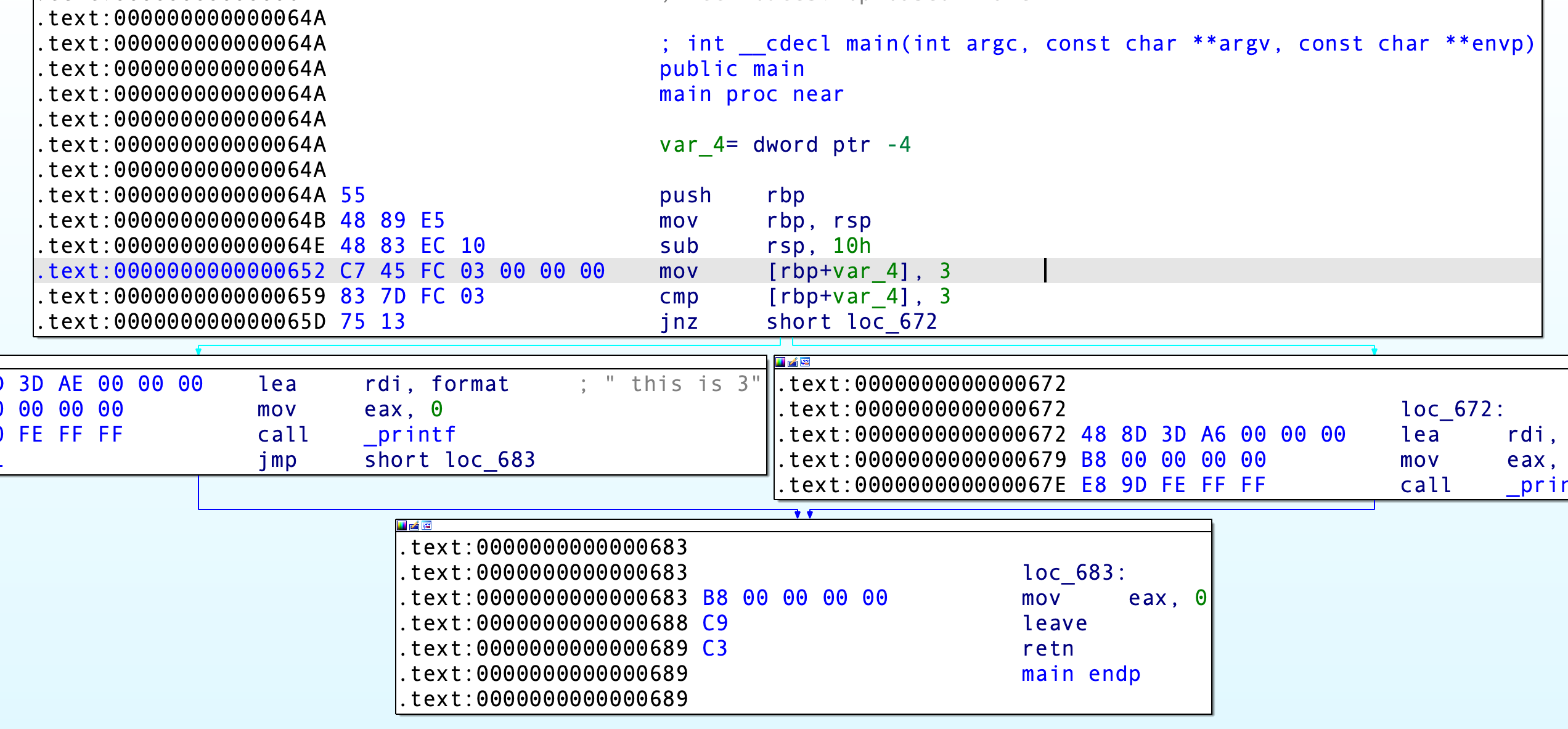
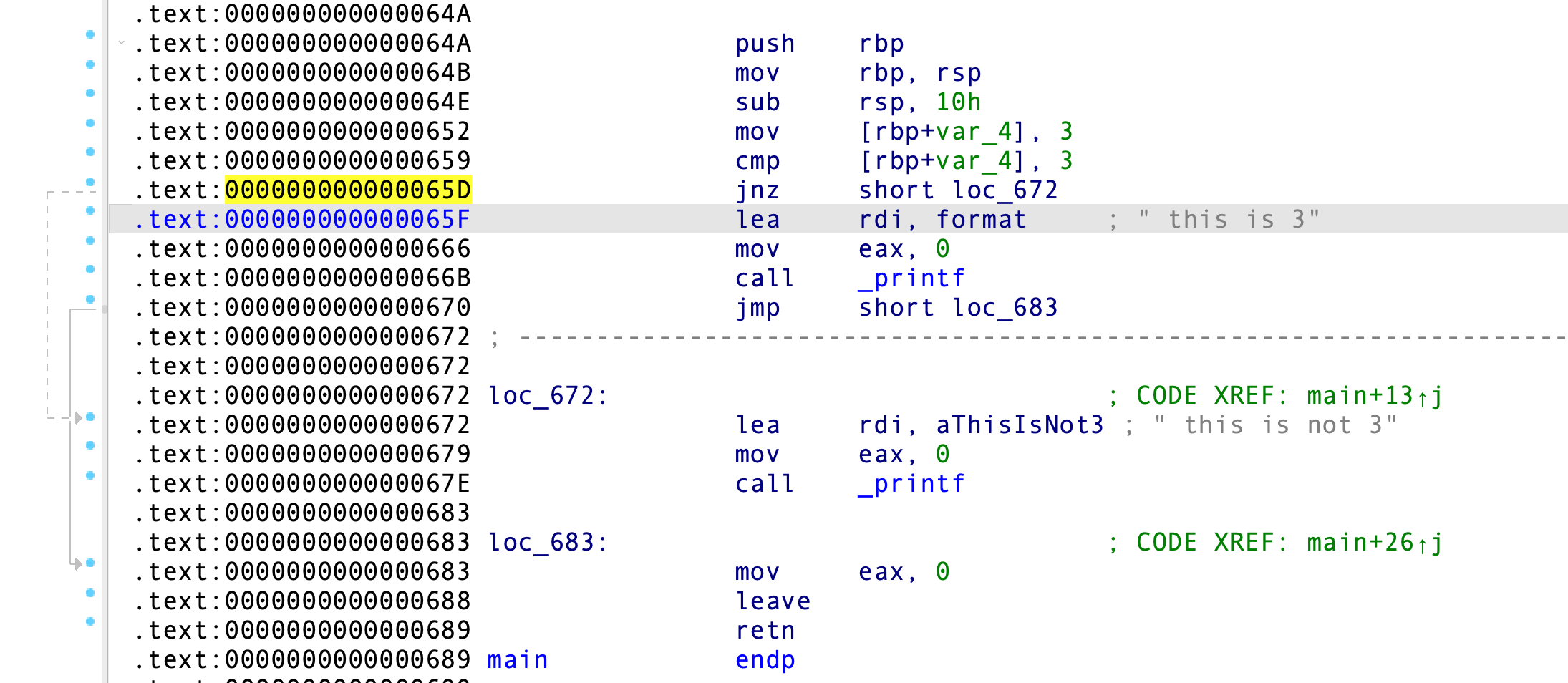
识别循环
for和while循环差距不大
for循环的4个组件
– 初始化、比较、执行指令、递增或递减
1 2 3 4 5 6 7 8 9 10 11 12
| #include <stdio.h>
int main() { int n = 0; for(int i=1;i<101;i++) n += i; printf("the sum is: %d\n",n); return 0;
}
|
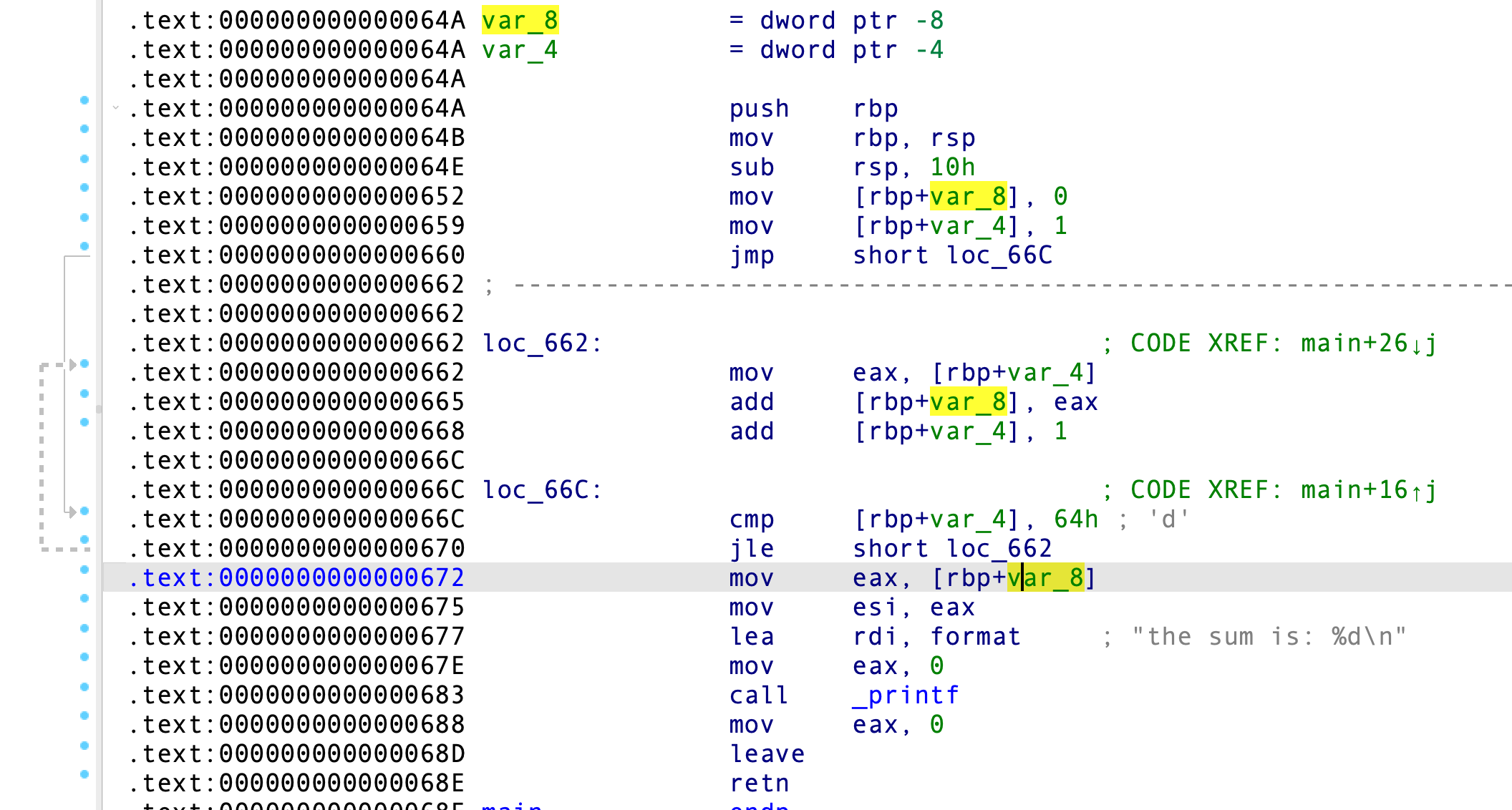
识别结构体
问题
一开始执行的是什么玩意??