c语言回炉重造-指针与数组
回炉重造, 还是通过gdb调试一下,理解的比较好, 多写代码多调试!
指针与地址初探
1 |
|
gcc -g main.c
ip是一个指针,在第六行,把x的地址赋给了指针,所以ip的值就是x的地址,ip本身就是一个值,也有自己的地址,
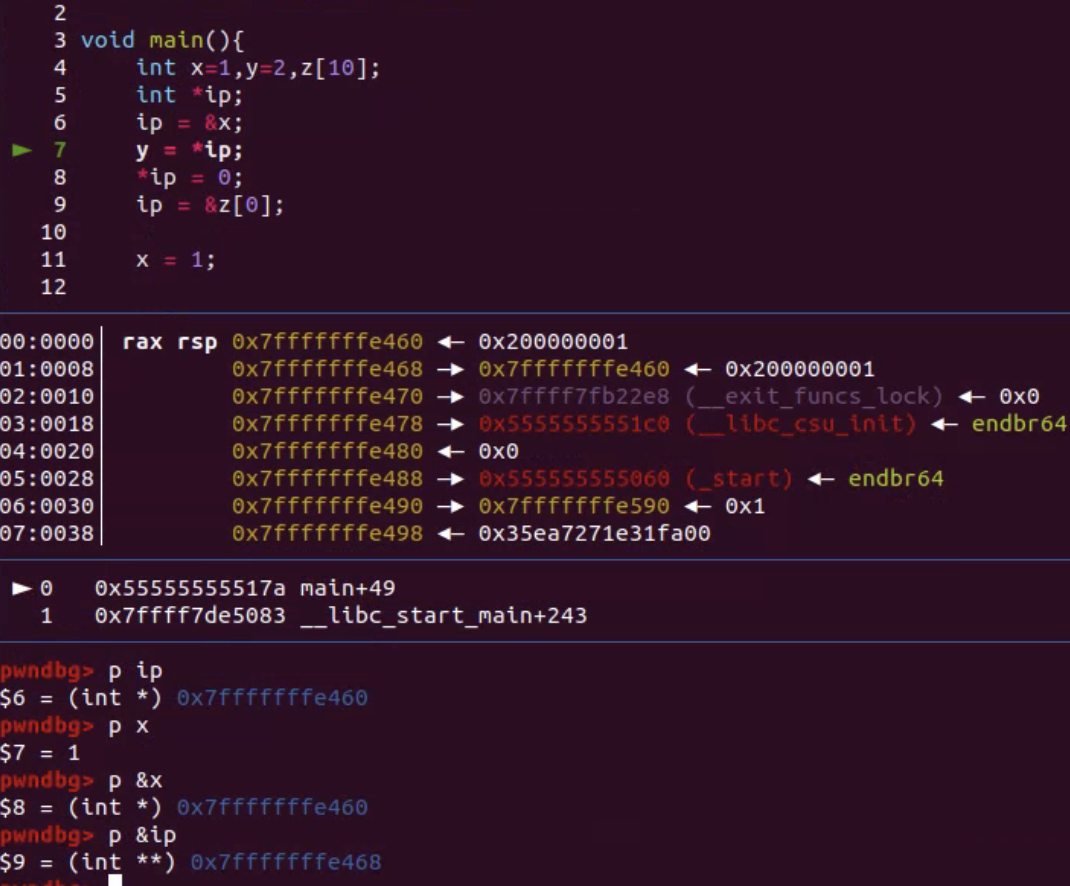
感觉这种未初始化的数组或许能做信息泄露?
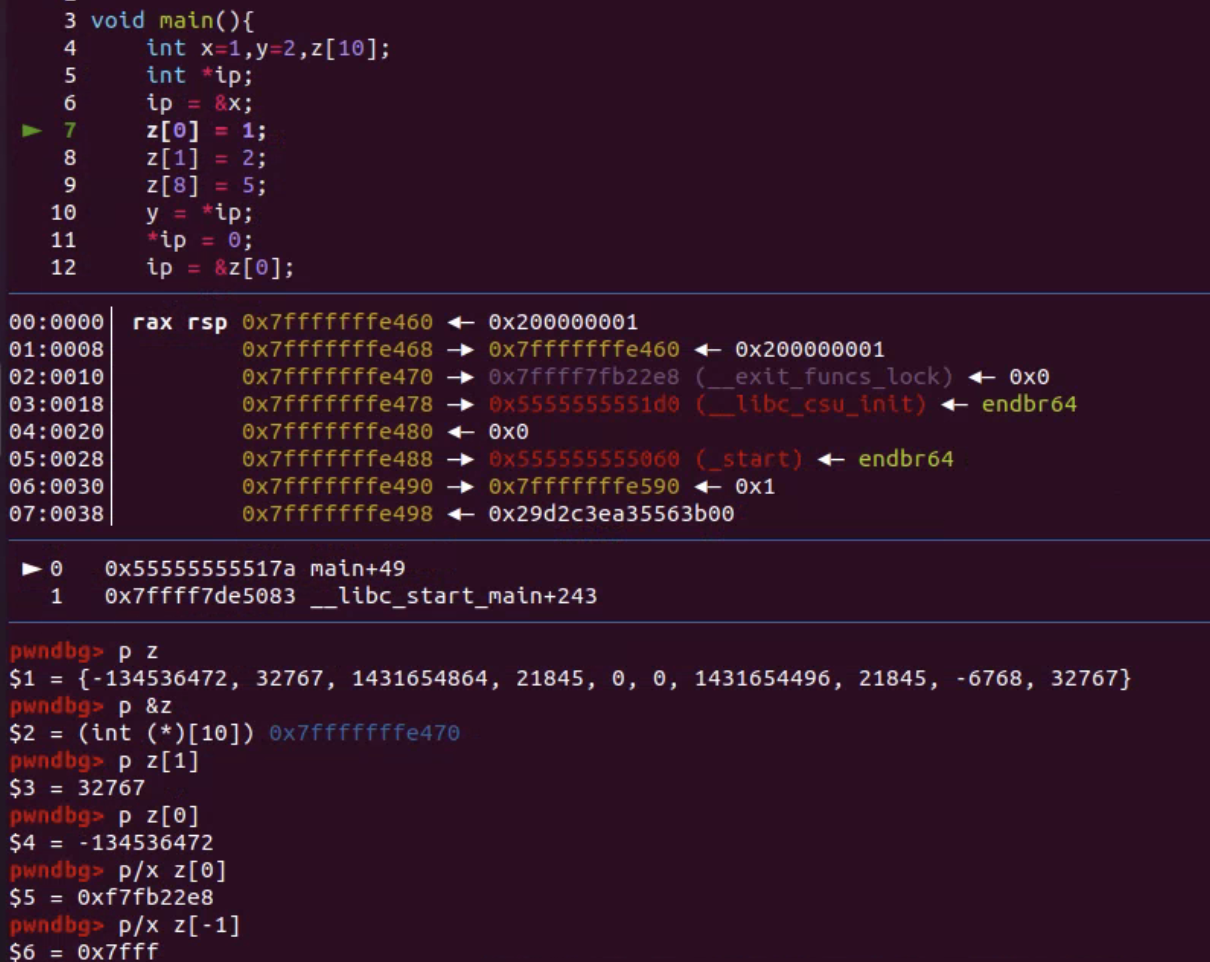
指针与函数参数
函数传递指针,实现赋值. 主要是getint函数,获取任意值,然后转换成数值,int类型的数值 而不是char类型的数值
1 |
|
关于 c-‘0’的问题
1 | for (*pn=0; isdigit(c); c=getch()) |
输入12345, ascii的话就是49 50 51 52 53
问题在于, 对于getchar()得到的数值,会是ascii码, 而直接赋值得到的是内存中数值,所以需要这么一个转换
1 |
|
虽然但是,a和b还是不一样的,a占用4个字节,b占用一个字节
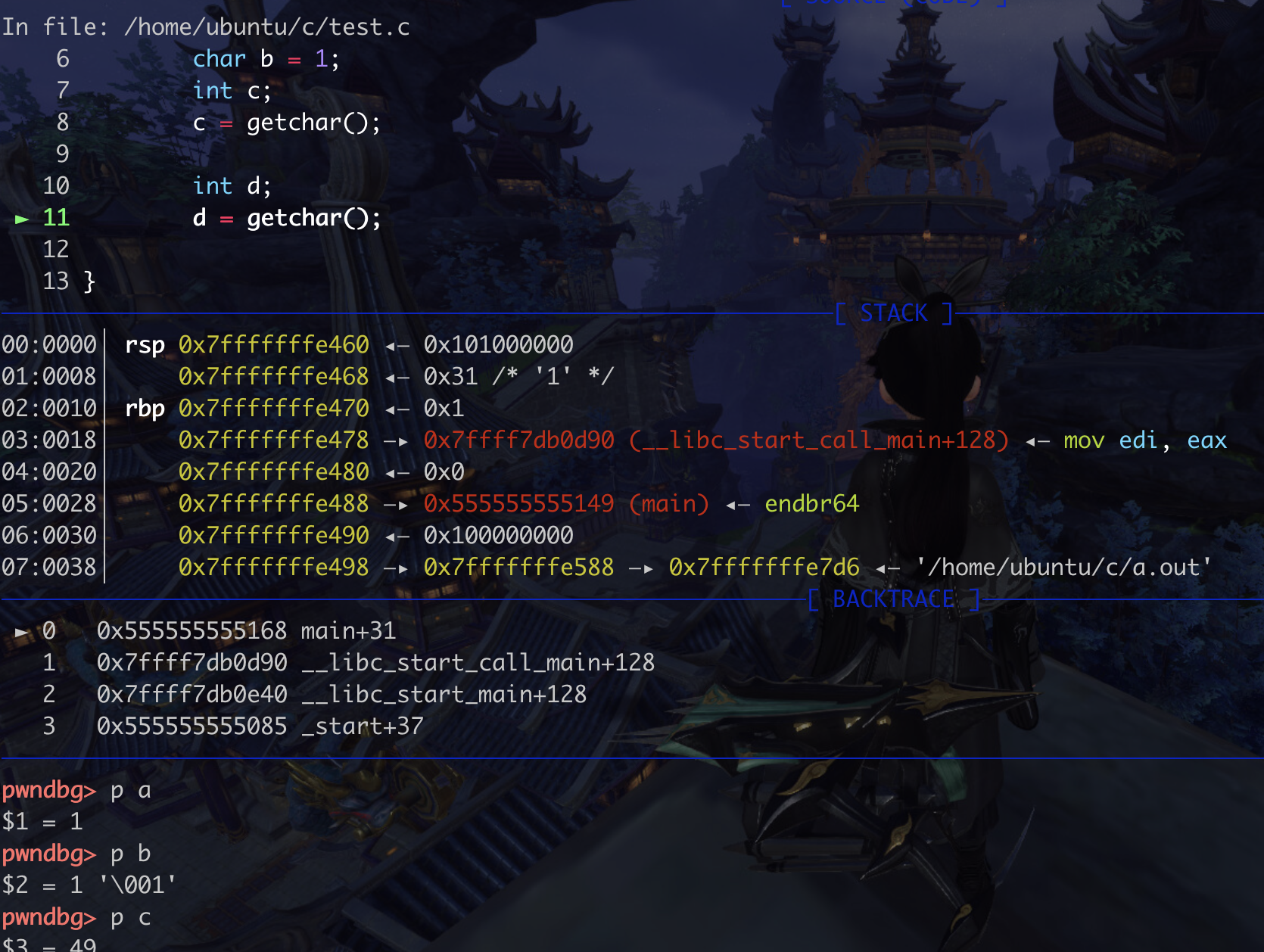
虽然但是,a和b还是不一样的,a占用4个字节,b占用一个字节
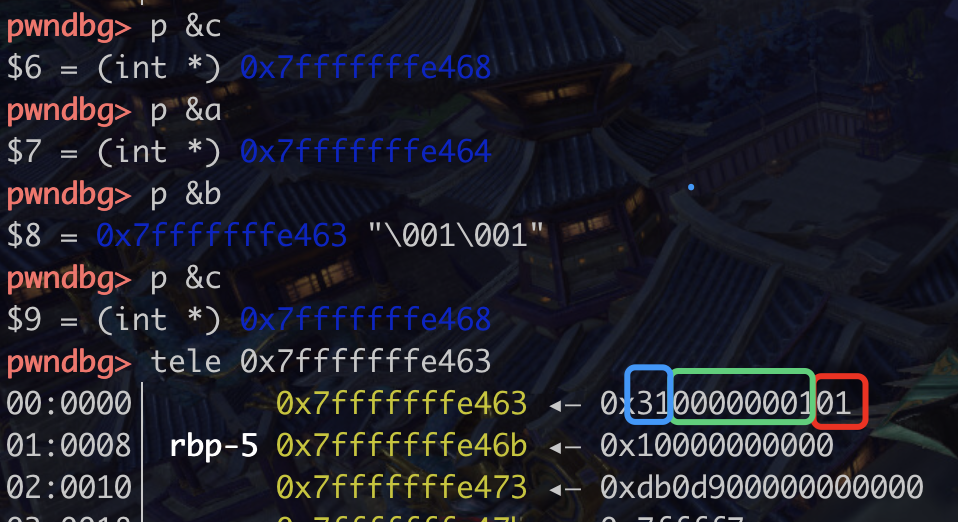
strlen 注意, 参数处的 *s 和 for循环里的 *s 是两个含义, 一个是代表定义指针, 一个是取指针指向的地址的值,差了两层
1 | int strlen(char *s) |
版本2, 数组的尾元素+1 (结束\0) 减头元素就是长度,
1 | int strlen(char *s) |
理解地址算数运算: 一个简单的存储分配程序
1 |
|
strcpy的实现
实现把指针t指向的字符串复制到指针s指向的位置, 但是不能用s=t,因为这个只是拷贝了指针,并没有复制字符串,
数组方法实现:
1 | void strcpy(char *s, char *t) |
指针方法实现:
1 | void strcpy(char *s, char *t) |
经验丰富的程序员更喜欢编写成一下形式:
1 | void strcpy(char *s,char *t) |
还可以进一步缩写,因为和0比较是多余的,while循环的条件本身就需要非0
1 | void strcpy(char *s,char *t) |
strcmp的实现
1 | int strcmp(char *s,char *t) |
用指针来实现
1 | int strcmp(char *s,char *t) |
指针数组排序
1 |
|
基础快速排序
1 |
|
多维数组
1 |
|
命令行参数 echo
第一个版本将argv看成一个字符指针数组
1 |
|
因为argv是一个指向指针数组的指针,所以,可以通过指针而非数组下标的方式处理命令行参数.
第二个版本是在对argv进行自增运算、对argc进行自减运算的基础上实现的(argv是一个指向char类型的指针的指针)
1 |
|
模式查找程序
4.1节 内置了查找模式
将输入中包含特定“模式”或字符串的各行打印出来(grep的特例相当于)
1 |
|
5.10 增强版 实现find功能,打印与第一个参数指定的模式匹配的行
1 |
|
再次改进
1 |
|
函数指针
1 |
|
(void **)是啥, 将指针数组转换成 指向指针数组的指针类型
复杂声明
程序dcl是啥
1 | char **argv |
q
能不能用gdb显示变量信息呢,结构信息等,比如char和int的区别
EOF的话,包括哪些