第二章 面向过程
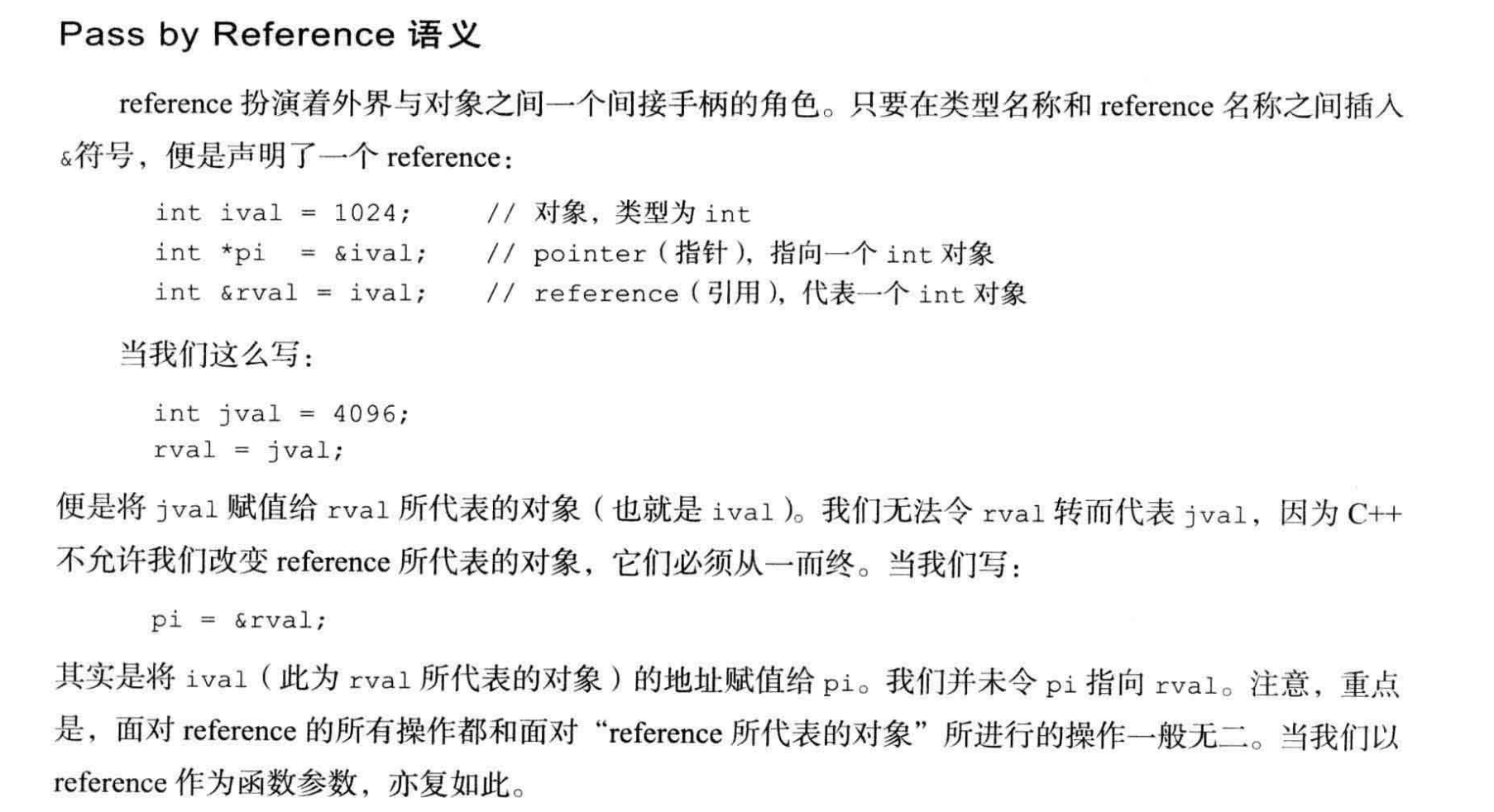
传参数 其实除了传递值本身,另外的其实有两种,一种是传递地址(pointer),一种是传递reference
???

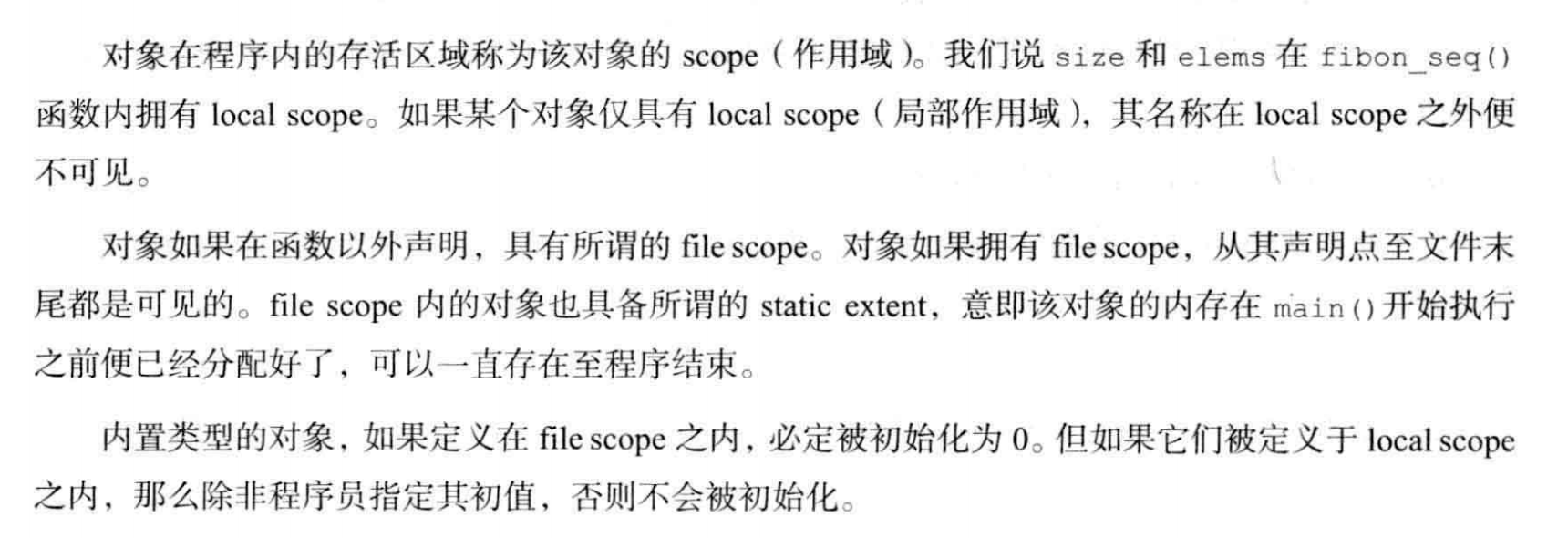
inline 性能和可读性
重载机制: 将一组实现代码不同但工作内容相似的函数加以重载,可以让函数用户更容易使用这些函数,如果没有重载机制,就需要为每个函数提供不同的名称
模版函数: 就是一些函数,功能一样,只是参数类型不一样,每一个都重写就很麻烦,模版函数可以实现将参数类型推迟绑定,绑定后生成一份函数实例
模版函数也可以再进行重载
函数指针有点晕,看看c那本书
章节2练习
练习2.1
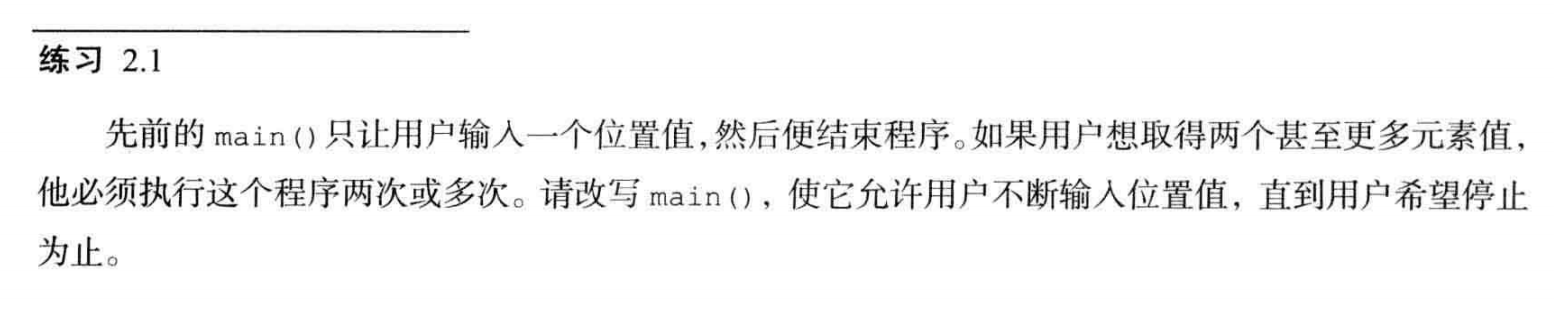
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| #include <iostream>
using namespace std;
bool fibon_elem(int,int &); int main() { int pos,elem; while(1){ cout << "please input a position,exit:-1 "; cin >> pos; if (pos == -1) return 0; if (fibon_elem(pos,elem)) cout << "element # " << pos << " is " << elem <<endl; else cout << "sorry, could not calculate element # "<< pos <<endl; }} bool fibon_elem(int pos,int &elem) { if (pos <=0 || pos >1024) { elem=0;return false;} elem =1; int n_1 = 1,n_2=1; for (int ix=3;ix<=pos;++ix) { elem = n_2+n_1; n_1 = n_2; n_2 = elem; } return true; }
|
练习2.2
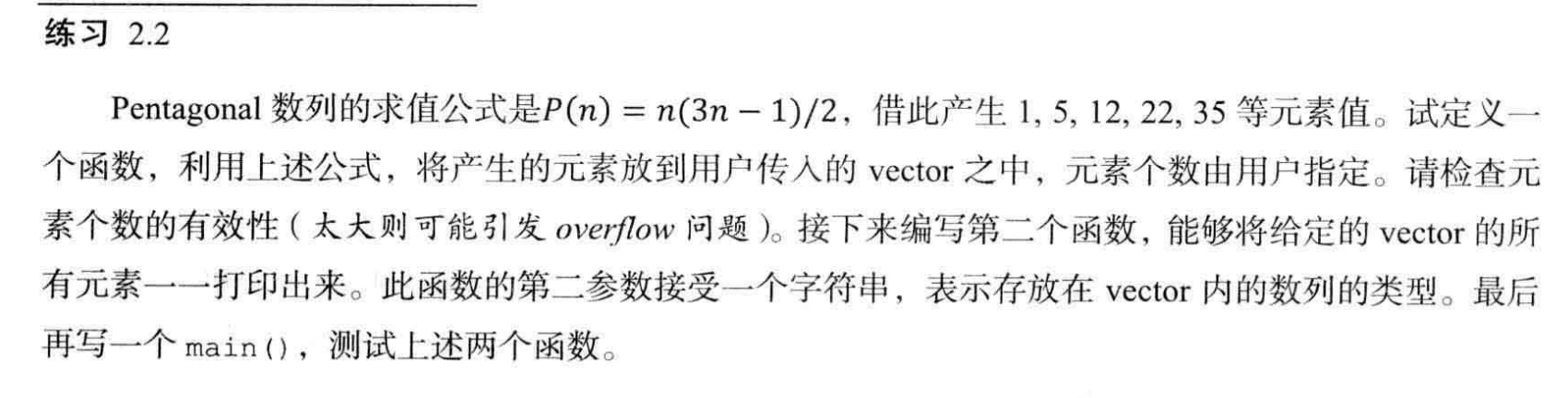
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| #include <iostream> #include <vector> using namespace std;
void add(vector<int> &vec,int num); void display(const vector<int> &vec);
int main() { vector<int> vec(0); while(1){ cout << "please input the num you want,exit:-1" << endl; int num; cin >> num; if (num == -1) exit(-1); add(vec,num); display(vec); } return 0; }
void add(vector<int> &vec,int num) { if (num<=0 || num > 1024) { cerr << "warning:num is wrong" << endl; exit(-1); } int pn=0; for (int ix=vec.size()+1;ix<=num;ix++) { pn = (ix*(3*ix - 1))/2; vec.push_back(pn); } } void display(const vector<int> &vec) { for (int ix=0;ix < vec.size(); ++ix) { cout << vec[ix] << ' ' ; } cout << endl; }
|
练习2.3

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| #include <iostream> #include <vector> using namespace std;
inline bool is_size_ok(int size); void add(vector<int> &vec,int size); void display(const vector<int> &vec);
int main() { vector<int> vec(0); cout << "please input the num you want" << endl; int size; cin >> size; add(vec,size); display(vec); return 0; }
inline bool is_size_ok(int size) { const int max_size = 1024; if(size <=0 || size > max_size) { cerr << "warning:num is wrong" << endl; return false; } return true; } void add(vector<int> &vec,int size) { if (!is_size_ok(size)) exit; int pn=0; for (int ix=1;ix<=size;ix++) { pn = (ix*(3*ix - 1))/2; vec.push_back(pn); } }
void display(const vector<int> &vec) { for (int ix=0;ix < vec.size(); ++ix) { cout << vec[ix] << ' ' ; } cout << endl; }
|
答案给的版本翻译过来
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55
| #include <iostream> #include <vector> using namespace std;
inline bool add(vector<int> &vec,int size); void display(const vector<int> &vec); void really_add(vector<int> &vec,int size); int main() { vector<int> vec(0); cout << "please input the num you want" << endl; int size; cin >> size; if(add(vec,size)) display(vec); return 0; }
inline bool add(vector<int> &vec,int size) { const int max_size = 1024; if(size <=0 || size > max_size) { cerr << "warning:num is wrong" << endl; return false; } if (vec.size() < size) really_add(vec,size); return true;
}
void really_add(vector<int> &vec,int size) { int pn=0; for (int ix=vec.size()+1;ix<=size;ix++) { pn = (ix*(3*ix - 1))/2; vec.push_back(pn); } }
void display(const vector<int> &vec) { for (int ix=0;ix < vec.size(); ++ix) { cout << vec[ix] << ' ' ; } cout << endl; }
|
练习2.4

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| #include <iostream> #include <vector> using namespace std;
void show(int num); inline bool is_size_ok(int size); vector<int>* store(); void add(int size);
int main() { cout << "please input the size you want" << endl; int size,num; cin >> size; add(size); cout << "please input the num you want" << endl; cin >> num; show(num);
return 0; }
inline bool is_size_ok(int size) { const int max_size = 1024; if(size <=0 || size > max_size) { cerr << "warning:num is wrong" << endl; return false; } return true; } vector<int>* store() { static vector<int> vecp; return &vecp; } void add(int size) { if (!is_size_ok(size)) exit; vector<int>* vecp= store(); vector<int>& vec = *vecp; int pn=0; for (int ix=vec.size();ix<=size;ix++) { pn = (ix*(3*ix - 1))/2; vec.push_back(pn); } }
void show(int num) { vector<int>* vecp= store(); vector<int>& vec = *vecp; cout << "this:" <<vec[num]<<endl; }
|
练习2.5
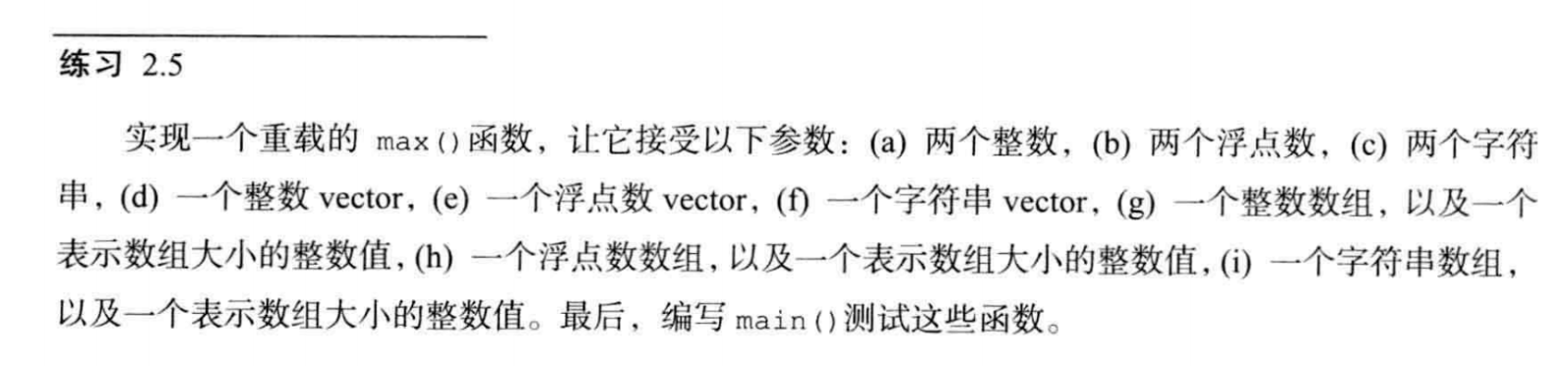
inline 的话,就不用再声明了?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75
| #include <string> #include <iostream> #include <vector> #include <algorithm> using namespace std;
int max(int a,int b); float max(float a,float b); string max(string a,string b); int max(vector<int> &vec); float max(vector<float> &vec); string max(vector<string> &vec); int max(int* a,int size); float max(float* a,int size); string max(string* a,int size);
int main() { string sarray[] = {"we","were","her","pride","of","ten"}; vector<string> svec(sarray,sarray+6); int iarray[] = {12,70,2,169,1,5,29}; vector<int> ivec(iarray,iarray+7); float farray[] = {2.5,24.8,18.7,4.1,23.9}; vector<float> fvec(farray,farray+5); int imax = max(max(ivec),max(iarray,7)); float fmax = max(max(fvec),max(farray,5)); string smax = max(max(svec),max(sarray,6)); cout << "imax should be 169 -- found: " << imax << '\n' << "fmax should be 24.8 -- found: " << fmax << '\n' << "smax should be were -- found: " << smax << '\n'; }
int max(int a,int b) { return a>b? a:b; } float max(float a,float b) { return a>b? a:b; } string max(string a,string b) { return a>b? a:b; } int max(vector<int> &vec) { return *max_element(vec.begin(),vec.end()); } float max(vector<float> &vec) { return *max_element(vec.begin(),vec.end()); } string max(vector<string> &vec) { return *max_element(vec.begin(),vec.end()); }
int max(int* arr,int size){ return *max_element(arr,arr+size); } float max(float* arr,int size){ return *max_element(arr,arr+size); } string max(string* arr,int size){ return *max_element(arr,arr+size); }
|
在写这个程序的时候遇到了很多奇奇怪怪的小问题,都是对很多地方理解不到位,有时间了慢慢总结.
练习2.6

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| #include <string> #include <iostream> #include <vector> #include <algorithm> using namespace std;
template <typename Type> inline Type max1(Type a,Type b) { return a>b? a:b; } template <typename elemType> inline elemType max1(const vector<elemType> &vec) { return *max_element(vec.begin(),vec.end()); }
template <typename arrayType> inline arrayType max1(const arrayType *arr,int size) { return *max_element(arr,arr+size); }
int main() { string sarray[] = {"we","were","her","pride","of","ten"}; vector<string> svec(sarray,sarray+6); int iarray[] = {12,70,2,169,1,5,29}; vector<int> ivec(iarray,iarray+7); float farray[] = {2.5,24.8,18.7,4.1,23.9}; vector<float> fvec(farray,farray+5); int imax = max1(max1(ivec),max1(iarray,7)); float fmax = max1(max1(fvec),max1(farray,5)); string smax = max1(max1(svec),max1(sarray,6)); cout << "imax should be 169 -- found: " << imax << '\n' << "fmax should be 24.8 -- found: " << fmax << '\n' << "smax should be were -- found: " << smax << '\n'; }
|
这里出现了一个奇怪的错误,网友也有很多遇到的
1 2
| 1.c:38:41: error: call of overloaded ‘max(int, int)’ is ambiguous 38 | int imax = max(max(ivec),max(iarray,7));
|
https://segmentfault.com/q/1010000019322724